- MongoDB CRUD Operations >
- Query Documents >
- Query an Array
Query an Array¶
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
This page provides examples of query operations on array fields using the
db.collection.find()
method in the
mongo
shell. The examples on this page use the
inventory
collection. To populate the inventory
collection, run the following:
This page provides examples of query operations on array fields using
MongoDB Compass. The examples on this
page use the inventory
collection. Populate the
inventory
collection with the following documents:
This page provides examples of query operations on array fields using the
pymongo.collection.Collection.find()
method in the
PyMongo
Python driver. The examples on this page use the inventory
collection. To populate the inventory
collection, run the
following:
This page provides examples of query operations on array fields using the com.mongodb.client.MongoCollection.find method in the MongoDB Java Synchronous Driver.
Tip
The driver provides com.mongodb.client.model.Filters helper methods to facilitate the creation of filter documents. The examples on this page use these methods to create the filter documents.
The examples on this page use the inventory
collection. To populate the inventory
collection, run the
following:
This page provides examples of query operations on array fields using the
Collection.find() method in
the MongoDB Node.js Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on array fields using the
MongoDB\Collection::find()
method in the
MongoDB PHP Library.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on array fields using the
motor.motor_asyncio.AsyncIOMotorCollection.find()
method in the Motor
driver. The examples on this page use the inventory
collection. To populate the inventory
collection, run the
following:
This page provides examples of query operations on array fields using the com.mongodb.reactivestreams.client.MongoCollection.find method in the MongoDB Java Reactive Streams Driver.
The examples on this page use the inventory
collection. To populate the inventory
collection, run the
following:
This page provides examples of query operations on array fields using the
MongoCollection.Find()
method in the
MongoDB C# Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on array fields using the
MongoDB::Collection::find() method
in the
MongoDB Perl Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on array fields using the
Mongo::Collection#find()
method in the
MongoDB Ruby Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
This page provides examples of query operations on array fields using the
collection.find() method
in the
MongoDB Scala Driver.
The examples on this page use the inventory
collection. To
populate the inventory
collection, run the following:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Match an Array¶
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
To specify equality condition on an array, use the query
document { <field>: <value> }
where <value>
is the
exact array to match, including the order of the elements.
To specify equality condition on an array, use the query
document { <field>: <value> }
where <value>
is the
exact array to match, including the order of the elements.
To specify equality condition on an array, use the query
document { <field>: <value> }
where <value>
is the
exact array to match, including the order of the elements.
To specify equality condition on an array, use the query
document eq( <field>, <value>)
where <value>
is
the exact array to match, including the order of the
elements.
To specify equality condition on an array, use the query
document { <field>: <value> }
where <value>
is the
exact array to match, including the order of the elements.
To specify equality condition on an array, use the query
document [ <field> => <value> ]
where <value>
is the
exact array to match, including the order of the elements.
To specify equality condition on an array, use the query
document { <field>: <value> }
where <value>
is the
exact array to match, including the order of the elements.
To specify equality condition on an array, use the query
document eq( <field>, <value>)
where <value>
is
the exact array to match, including the order of the
elements.
To specify equality condition on an array, construct a filter using the Eq method:
<value>
is the exact array to match, including the
order of the elements.
To specify equality condition on an array, use the query
document { <field> => <value> }
where <value>
is the
exact array to match, including the order of the elements.
To specify equality condition on an array, use the query
document { <field> => <value> }
where <value>
is the
exact array to match, including the order of the elements.
To specify equality condition on an array, use the query
document equal( <field>, <value> )
where <value>
is
the exact array to match, including the order of the
elements.
The following example queries for all documents where the field tags
value is an array with exactly two elements, "red"
and "blank"
,
in the specified order:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
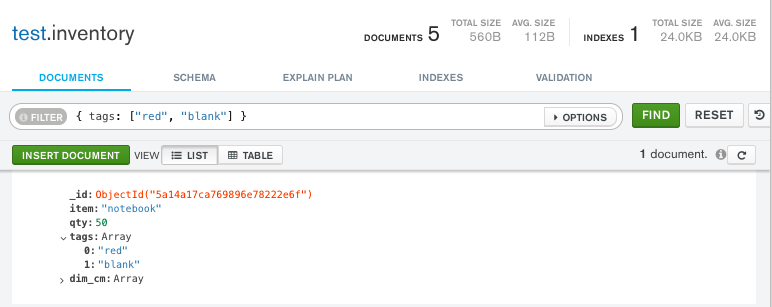
If, instead, you wish to find an array that contains both the elements
"red"
and "blank"
, without regard to order or other elements in
the array, use the $all
operator:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
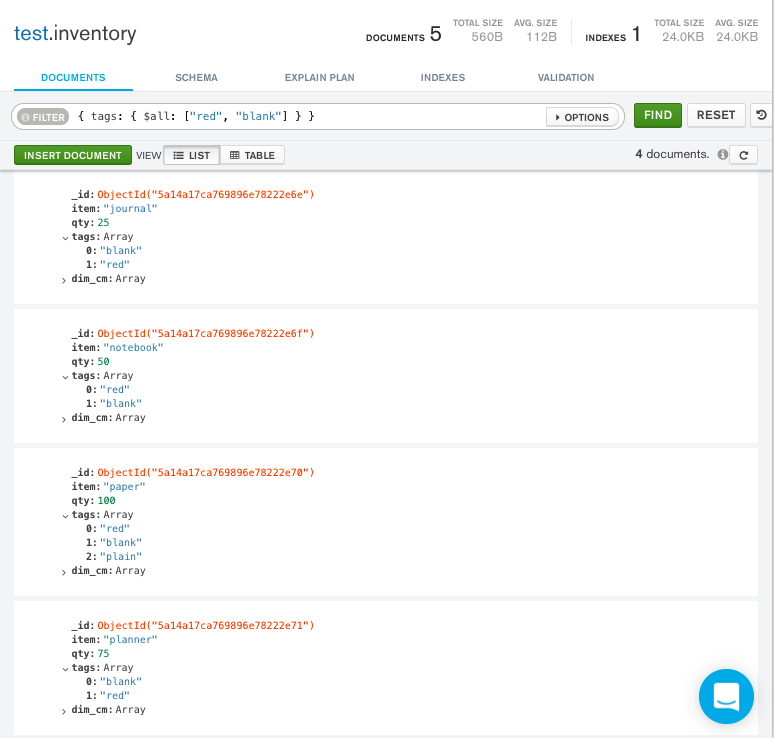
Query an Array for an Element¶
- Mongo Shell
- Python
- Java (Sync)
- Node.js
- PHP
- Other
To query if the array field contains at least one element
with the specified value, use the filter
{ <field>: <value> }
where <value>
is the element value.
To query if the array field contains at least one element
with the specified value, use the filter
{ <field>: <value> }
where <value>
is the element value.
To query if the array field contains at least one element
with the specified value, use the filter
eq( <field>, <value>)
where <value>
is the element value.
To query if the array field contains at least one element
with the specified value, use the filter
{ <field>: <value> }
where <value>
is the element value.
To query if the array field contains at least one element
with the specified value, use the filter
[ <field> => <value> ]
where <value>
is the element value.
To query if the array field contains at least one element
with the specified value, use the filter
{ <field>: <value> }
where <value>
is the element value.
To query if the array field contains at least one element
with the specified value, use the filter
eq( <field>, <value>)
where value is the element value.
To query if the array field contains at least one element with the specified value, construct a filter using the Eq method:
<value>
is the element value to match.
To query if the array field contains at least one element with the specified value, use the filter
{ <field> => <value> }
where value is the element value.
To query if the array field contains at least one element
with the specified value, use the filter
{ <field> => <value> }
where <value>
is the element value.
To query if the array field contains at least one element
with the specified value, use the filter
equal( <field>, <value> )
where <value>
is the element value.
The following example queries for all documents where tags
is an
array that contains the string "red"
as one of its elements:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
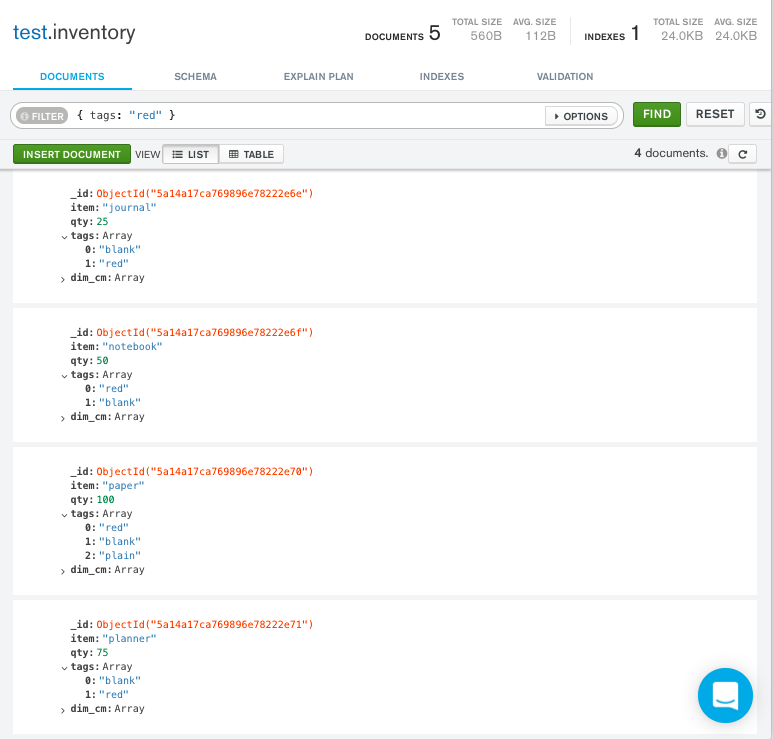
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
To specify conditions on the elements in the array field, use query operators in the query filter document:
To specify conditions on the elements in the array field, use query operators in the query filter document:
To specify conditions on the elements in the array field, use query operators in the query filter document:
To specify conditions on the elements in the array field, use query operators in the query filter document. For example:
To specify conditions on the elements in the array field, use query operators in the query filter document:
To specify conditions on the elements in the array field, use query operators in the query filter document:
To specify conditions on the elements in the array field, use query operators in the query filter document:
To specify conditions on the elements in the array field, use query operators in the query filter document. For example:
To specify conditions on the elements in the array field, use query operators in the query filter document. For example:
To specify conditions on the elements in the array field, use query operators in the query filter document:
To specify conditions on the elements in the array field, use query operators in the query filter document:
To specify conditions on the elements in the array field, use query operators in the query filter document:
For example, the following operation queries for all documents where the array
dim_cm
contains at least one element whose value is greater than
25
.
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:

Specify Multiple Conditions for Array Elements¶
When specifying compound conditions on array elements, you can specify the query such that either a single array element meets these condition or any combination of array elements meets the conditions.
Query an Array with Compound Filter Conditions on the Array Elements¶
The following example queries for documents where the dim_cm
array
contains elements that in some combination satisfy the query
conditions; e.g., one element can satisfy the greater than 15
condition and another element can satisfy the less than 20
condition, or a single element can satisfy both:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
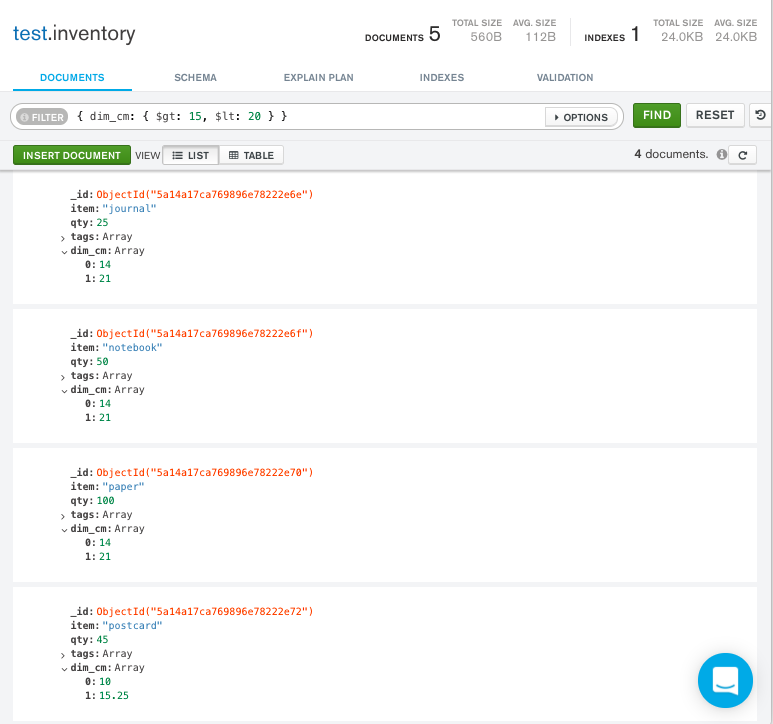
Query for an Array Element that Meets Multiple Criteria¶
Use $elemMatch
operator to specify multiple criteria on the
elements of an array such that at least one array element satisfies all
the specified criteria.
The following example queries for documents where the dim_cm
array
contains at least one element that is both greater than ($gt
)
22
and less than ($lt
) 30
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
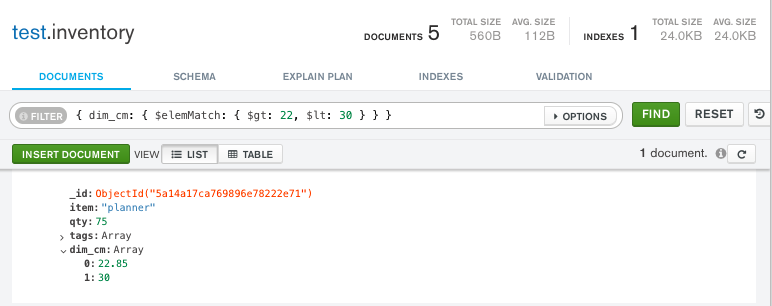
Query for an Element by the Array Index Position¶
Using dot notation, you can specify query conditions for an element at a particular index or position of the array. The array uses zero-based indexing.
Note
When querying using dot notation, the field and nested field must be inside quotation marks.
The following example queries for all documents where the second
element in the array dim_cm
is greater than 25
:
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:
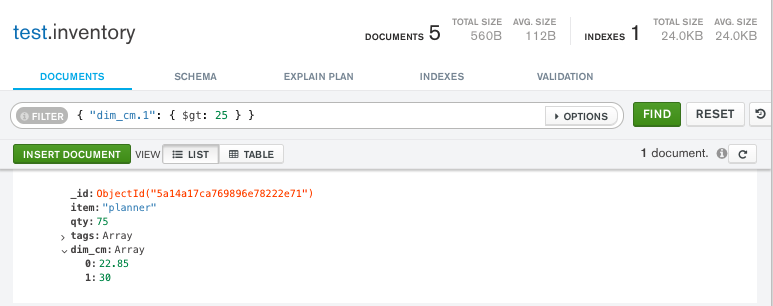
Query an Array by Array Length¶
Use the $size
operator to query for arrays by number of
elements. For example, the following selects documents where the array
tags
has 3 elements.
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
Copy the following filter into the Compass query bar and click Find:

Additional Query Tutorials¶
For additional query examples, see: